If you haven’t used Google Analytics before, I can highly recommend you take a look. It’s a free service that allows you to get detailed traffic and usage information for your website. You don’t need to be paying for (or earning money from) Google via its advertising business, although Analytics does integrate with those services too. Your web site will most likely already have a log analysis solution (e.g. Webalizer) that outputs a pretty summary of visitors/hits/etc. of the traffic on your site. Analytics gives you a lot more information, although its easy to get at.
The really exciting thing for RIAs however, is that you can integrate them with Google Analytics, which your existing traffic analysis solution probably doesn’t do. You see, most traffic analysis tools work by keeping track fo the most commonly-requested URIs on a site. That’s fine for a traditional HTML-based site like a blog. With a RIA (such as an OpenLaszlo, Flash, AJAX, Flex, etc. application) the only things that would show up on your traffic stats would be the request for the app (e.g. SWF) itself, and the web services that it calls. Those web service calls may give you some clue as to users’ behavior, but you won’t see any user action that doesn’t involve an HTTP request. For example, if you have a tabslider component, you may want to know how often a particular tab is opened, but if the opening of that tab element doesn’t result in a request, the logs won’t be much use.
Here's how Google Analytics deals with this problem: Every HTML page on your site (including the wrapper page for an OpenLaszlo application) embeds a small piece of JavaScript (hosted by Google). That's how Google keeps track of your visitors in general. It also provides a browser JavaScript function you can call that takes an arbitrary string and logs that to your Analyitcs account. You can create whatever name you want. It'll show up in the Content Overview section of the report, along with other HTML pages that were accessed:
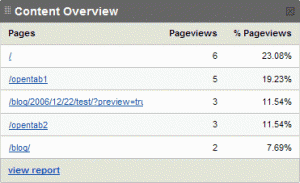
As far as the LZX code is concerned, it's actually quite simple. You will need to call a Google browser JavaScript method called pageTracker._trackPageView() whenever you want to register a user action from within your OpenLaszlo application. Since _trackPageView is a browser JavaScript method, you need to use the LzBrowser.loadJS() method to call it. (That's for OpenLaszlo 4.1 and below; it's lz.Browser in OpenLaszlo 4.2):
<canvas proxied="false"> <tabslider width="400" height="300"> <tabelement>First Tab <handler name="onopenstart"> LzBrowser.loadJS("pageTracker._trackPageview('/opentab1')"); </handler> <text fontsize="16"> Thank you for looking at the first tab. </text> </tabelement> <tabelement>Second Tab <handler name="onopenstart"> LzBrowser.loadJS("pageTracker._trackPageview('/opentab2')"); </handler> <text fontsize="16"> Thank you for looking at the second tab. </text> </tabelement> <tabelement>Third Tab <handler name="onopenstart"> LzBrowser.loadJS("pageTracker._trackPageview('/opentab3')"); </handler> <text fontsize="16"> Thank you for looking at the third tab. </text> </tabelement> </tabslider> </canvas>
Remember that you will have needed to embed the Google JavaScript include in your HTML wrapper page in order to access this method first.